Nota
Fare clic qui per scaricare il codice di esempio completo
Stili di scatole personalizzate #
Questo esempio illustra l'implementazione di un file BoxStyle
. ConnectionStyle
Le s e le s personalizzate ArrowStyle
possono essere definite in modo simile.
from matplotlib.patches import BoxStyle
from matplotlib.path import Path
import matplotlib.pyplot as plt
Gli stili di casella personalizzati possono essere implementati come una funzione che accetta argomenti che specificano sia una casella rettangolare che la quantità di "mutazione" e restituisce il percorso "mutato". La firma specifica è quella di
custom_box_style
seguito.
Qui restituiamo un nuovo percorso che aggiunge una forma a "freccia" a sinistra del riquadro.
Lo stile della casella personalizzata può quindi essere utilizzato passando
a .bbox=dict(boxstyle=custom_box_style, ...)
Axes.text
def custom_box_style(x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box around
it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Mutation reference scale, typically the text font size.
"""
# padding
mypad = 0.3
pad = mutation_size * mypad
# width and height with padding added.
width = width + 2 * pad
height = height + 2 * pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2), (x0, y0),
(x0, y0)],
closed=True)
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle=custom_box_style, alpha=0.2))
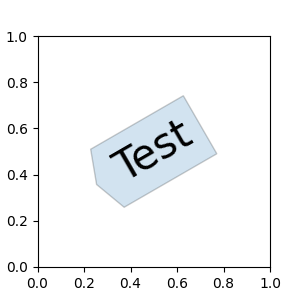
Text(0.5, 0.5, 'Test')
Allo stesso modo, gli stili box personalizzati possono essere implementati come classi che implementano
__call__
.
Le classi possono quindi essere registrate nel BoxStyle._style_list
dict, che consente di specificare lo stile della casella come una stringa,
. Tieni presente che questa registrazione si basa su API interne e pertanto non è ufficialmente supportata.bbox=dict(boxstyle="registered_name,param=value,...", ...)
class MyStyle:
"""A simple box."""
def __init__(self, pad=0.3):
"""
The arguments must be floats and have default values.
Parameters
----------
pad : float
amount of padding
"""
self.pad = pad
super().__init__()
def __call__(self, x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box
around it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Reference scale for the mutation, typically the text font size.
"""
# padding
pad = mutation_size * self.pad
# width and height with padding added
width = width + 2.*pad
height = height + 2.*pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2.), (x0, y0),
(x0, y0)],
closed=True)
BoxStyle._style_list["angled"] = MyStyle # Register the custom style.
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle="angled,pad=0.5", alpha=0.2))
del BoxStyle._style_list["angled"] # Unregister it.
plt.show()
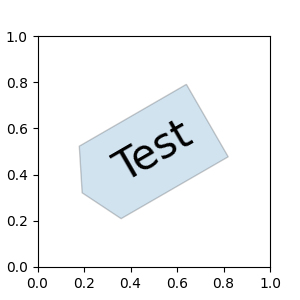